Puppeteer
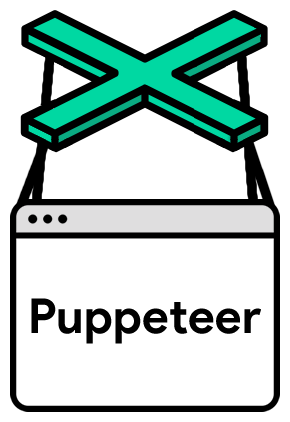
Puppeteer 是一个 JavaScript 库,它提供了一个高级 API 来通过 开发工具协议 或 WebDriver 双向 控制 Chrome 或 Firefox。Puppeteer 默认在无头(无可见 UI)模式下运行
¥Puppeteer is a JavaScript library which provides a high-level API to control Chrome or Firefox over the DevTools Protocol or WebDriver BiDi. Puppeteer runs in the headless (no visible UI) by default
开始使用 | API | 常见问题 | 贡献 | 故障排除
¥Get started | API | FAQ | Contributing | Troubleshooting
安装
¥Installation
- npm
- Yarn
- pnpm
npm i puppeteer # Downloads compatible Chrome during installation.
npm i puppeteer-core # Alternatively, install as a library, without downloading Chrome.
yarn add puppeteer # Downloads compatible Chrome during installation.
yarn add puppeteer-core # Alternatively, install as a library, without downloading Chrome.
pnpm add puppeteer # Downloads compatible Chrome during installation.
pnpm add puppeteer-core # Alternatively, install as a library, without downloading Chrome.
示例
¥Example
import puppeteer from 'puppeteer';
// Or import puppeteer from 'puppeteer-core';
// Launch the browser and open a new blank page
const browser = await puppeteer.launch();
const page = await browser.newPage();
// Navigate the page to a URL.
await page.goto('https://developer.chrome.com/');
// Set screen size.
await page.setViewport({width: 1080, height: 1024});
// Type into search box.
await page.locator('.devsite-search-field').fill('automate beyond recorder');
// Wait and click on first result.
await page.locator('.devsite-result-item-link').click();
// Locate the full title with a unique string.
const textSelector = await page
.locator('text/Customize and automate')
.waitHandle();
const fullTitle = await textSelector?.evaluate(el => el.textContent);
// Print the full title.
console.log('The title of this blog post is "%s".', fullTitle);
await browser.close();